bind 함수란 ?
: this 값이 없을 때 강제로 this 값을 주입하는 방법이라고 할 수 있다.
여기서 this는 render 함수 안에서 해당 render 함수가 속한 컴포넌트 자체를 가리킨다.
bind 함수를 이해하기 위해 예시를 들어보겠음
App.js
class App extends Component {
constructor(props) {
super(props);
this.state = {
mode:'read',
subject:{title:'WEB', sub:'World Wide Web!'}
}
}
render() {
console.log("render", this);
}
}
console.log("render", this)로 this값을 콘솔로 출력하고, 이 코드에서 this는 App 컴포넌트라고 할 수 있다.
그래서 코드를 실행해보면 console창에
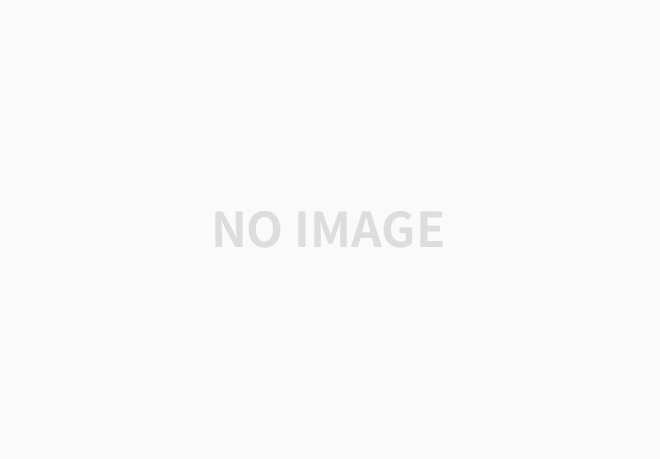
이런 식으로 뜨는 것을 확인해볼 수 있다.
그런데 여기서 코드를 조금 수정해보자.
class App extends Component {
constructor(props) {
super(props);
this.state = {
mode:'read',
subject:{title:'WEB', sub:'World Wide Web!'}
}
}
render() {
console.log("render", this);
return (
<header>
<h1><a href='/' onClick={function(e) {
console.log("event in", this);
}}>{this.state.subject.title}</a></h1>
</header>
)
}
}
이렇게 return 값에 <a> 태그를 넣고, <a> 태그를 클릭하면 function이 실행된다.
function은 this값을 콘솔창으로 출력함.
근데 이 코드를 실행해보면
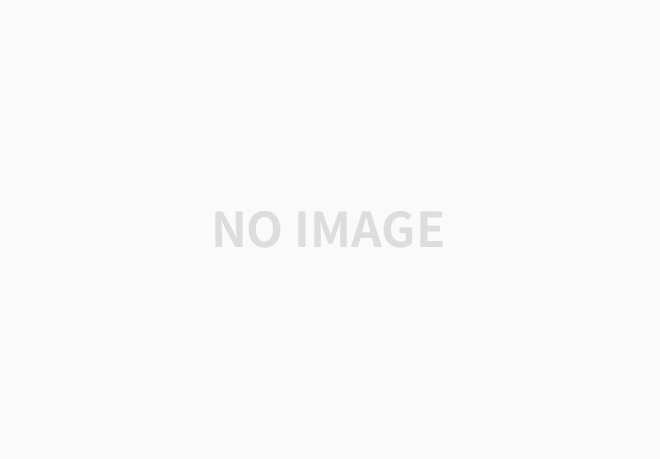
<a> 태그의 onClick 이벤트 처리 함수는 this가 아무것도 없기 때문에 이렇게 콘솔창에 출력된다.
그래서 함수 뒤에 .bind를 넣어주면 bind가 this값을 강제로 주입해 실행되게 해준다.
class App extends Component {
constructor(props) {
super(props);
this.state = {
mode:'read',
subject:{title:'WEB', sub:'World Wide Web!'}
}
}
render() {
console.log("render", this);
return (
<header>
<h1><a href='/' onClick={function(e) {
console.log("event in", this);
}.bind(this)}>{this.state.subject.title}</a></h1>
</header>
)
}
}
setState 함수
setState 함수는 말 그대로 state를 바꾸게 될 때 쓰이는 함수이다.
setState 함수를 쓰는 이유는 간단하다.
this.state 값을 직접 변경하면 리액트 입장에선 state 값이 바뀐지 모르기 때문이다.
this.state.mode = 'read';
이 코드를 실행하게 되면 오류없이 작동은 잘 된다.
하지만 이 코드를 가지는 function이 실행돼도 실제로 mode의 값이 변경되지 않는다.
그렇다고 this.state.mode의 값이 변하지 않은 건 아니다.
리액트가 모를 뿐...
그래서 setState 함수를 이용해 this.state값을 변경해야한다.
this.setState({mode : "welcome"});
정리
리액트는 내 말을 듣지 않는 바보다.
'Library | Framework > React' 카테고리의 다른 글
[React] 컴포넌트 이벤트 만들기 복습/응용 (0) | 2022.01.05 |
---|---|
[React] 컴포넌트 이벤트 만들기(onChangePage) (0) | 2022.01.05 |
[React] event, render 함수, state 값 변경 방법 (3) | 2022.01.03 |
[React] state, props, key props (1) | 2022.01.03 |
[React] React 프로젝트 생성, 컴포넌트화 (0) | 2021.12.28 |
댓글